Hello, in this article we will be looking at the filter() method, which is a javascript method frequently used by frontend developers in daily business life. As in my other javascript method blog posts, in this post, we will reinforce the topic by doing a standard simple examples with the filter method and then an example of how it is used in a React project. What is this filter javascript method without wasting time? Let’s learn how to use it…
- Creating a Project Structure with Next.js 13Recently the new version of Next.js, version 13, was released. In this content, I will create a Next.js project structure in accordance with the new version.
- How to make pagination in React without using npm package?I will be explaining how you can make pagination in your react projects using only Javascript and React Hooks without using an npm package like “react-paginate”.
- 2022 – Javascript Array Methods: filter()Hello, in this article we will be looking at the filter() method, which is a javascript method frequently used by frontend developers in daily business life. As in my other… Read more: 2022 – Javascript Array Methods: filter()
What is the Javascript Filter Method?
As the name suggests, it ensures that the data in the array we have is filtered by going through the desired process and a new array is created and returned. There will be no change on the main array. But it can be done if desired.?
Now let’s look at the usage structure of the filter method….
array.filter(function(currentValue, index, array), thisValue)
- currentValue : Denotes the value of the element being processed.
- index : Specifies the index value of the processed element.
- array : Specifies the array value being processed.
- thisValue : Specifies the this value to be used when the specified operation is running, that is, when the callback function is executed.
Important Warnings
- If no value is found in the array in the operation or if the given array is empty, the filter method will return an empty array.
Now let’s reinforce what we have learned by making examples about the filter method.
Simple Examples
Finding Words with Characters Greater than 6 in Array
const words = ['spray', 'limit', 'elite', 'exuberant', 'destruction', 'present'];
const result = words.filter(word => word.length > 6);
console.log(result);
// Output: Array ["exuberant", "destruction", "present"]
In the example above, there is an array named words that we created with const. Then we create another variable called resualt and determine its value by passing the words array through a filter operation. What exactly is being done in this process? We process each word to see if the number of characters is greater than 6. As a result of the process, the words exuberant, destruction and present with a character count greater than 6 are given as an output in an array. Let’s see how the same operation works on a data which is also an object. Actually there is not a big difference. We will just do the same operations on the object.
Filtering on Objects in Array
const words = [
{id: 1,"word":"spray"},
{id:2, "word":"limit"},
{id:3, "word":"elite"},
{id:4, "word":"exuberant"},
{id:5, "word":"destruction"},
{id:6, "word":"present"},
];
const result = words.filter(item => item.word.length > 6);
console.log(result);
In the console, we will again see an output like the one below.
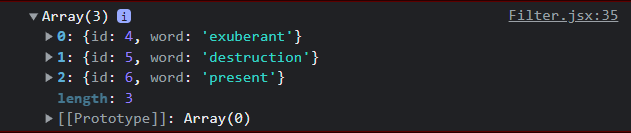
Filtering for Search Purposes
As a last simple example, if we want to create a function for searching in an array, let’s look at an example of how to do it.
const words = ['spray', 'limit', 'elite', 'exuberant', 'destruction', 'present'];
const search = keyword => words.filter(word => word.toLowerCase().includes(keyword.toLowerCase()));
console.log(search("es"));
According to the codes above, toLowerCase and includes methods appear, if I make a small definition about them.
- toLowerCase : Used to lowercase all characters in a string expression.
- includes : Tests if the specified value is in the array.
word => word.toLowerCase().includes(keyword.toLowerCase())
It may not be clear what exactly the above filter operation does. If I explain it in a simple way. word is our value that is subject to the filter operation. With toLowerCase, we convert all the characters of the word in the string value in the word value to lowercase. In the same way, we reduce the letters of the word in the string value that comes with the keyword. Then we search the word value for the keyword value with includes. If the result is true, that word value will be filtered and returned to us in the array. I hope this is clear. ?

Now when we call the search function with the “es” parameter and print it to the console screen, it should return us the two values in the red boxes as an array. Yes, as you can see below, the above coding works successfully. ?
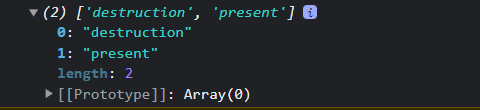
Now let’s take a look at how the filter method is used by frontend developers in daily business life…Now let’s take a look at how the filter method is used by frontend developers in daily business life…
Using Filter in a React Project
Filter method, like map method, is a javascript array method that is widely used by front end developers. If you have switched to a javascript library like React, Vue or Angular, I can say that it is impossible not to encounter the map or filter method. ? Here we will reinforce the use of the filter method with an example of how it is used in a react project.
Again, we will be using the Rest Api source “https://jsonplaceholder.typicode.com/“, which I used in my other javascript array methods posts. I will be going over the code in my previous find method blog post. I suggest you take a look there as well.
Now let’s make a filtering here. I want only the blog posts of the user with the username “Karianne” to be listed, how can I do this? Now let’s do the coding for this.
With the filter method, a new array will be created and we will map the object elements in this array to each element and get the output. Now I created a new function called dataFilter.
const dataFilter = (username) => {
return data.filter((item) => usernameValue(item.userId) === username);
}
Let me explain what exactly is being done in the function above. Our function receives a value called username. Then, by applying a filter operation to the data array, we ensure that the data that is equal to the username value we have sent and the username value returned from the usernameValue function is returned to us as an array.
In this way, when we change the data we print with the map in return to dataFilter, only the filtered data will be printed on the screen.
{dataFilter("Karianne")?.map((item) => {
return (
<div className="card-item" key={item.id}>
<span className="card-username">
{usernameValue(item.userId)}
</span>
<h2 className="card-title">{item.title}</h2>
<p className="card-description">{item.body}</p>
</div>
);
})}
When we want to print only the contents of “Karianne”, it will be enough to change the data part in return to dataFilter(“Karianne”). I will be sharing the complete filter codes with you below.
import { useEffect, useState } from "react";
const Filter = () => {
const [data, setData] = useState([]);
const [users, setUsers] = useState([]);
useEffect(() => {
fetch("https://jsonplaceholder.typicode.com/posts")
.then((response) => response.json())
.then((resPost) => {
setData(resPost);
});
fetch("https://jsonplaceholder.typicode.com/users")
.then((response) => response.json())
.then((resUsers) => {
setUsers(resUsers);
});
}, []);
const usernameValue = (id) => {
return users.find((user) => user.id === id).username;
};
const dataFilter = (username) => {
return data.filter((item) => usernameValue(item.userId) === username);
}
return (
<div id="content">
<h1>Filter</h1>
<div className="card">
{dataFilter("Karianne")?.map((item) => {
return (
<div className="card-item" key={item.id}>
<span className="card-username">
{usernameValue(item.userId)}
</span>
<h2 className="card-title">{item.title}</h2>
<p className="card-description">{item.body}</p>
</div>
);
})}
</div>
</div>
);
};
export default Filter;
Finally, let’s look at the output.
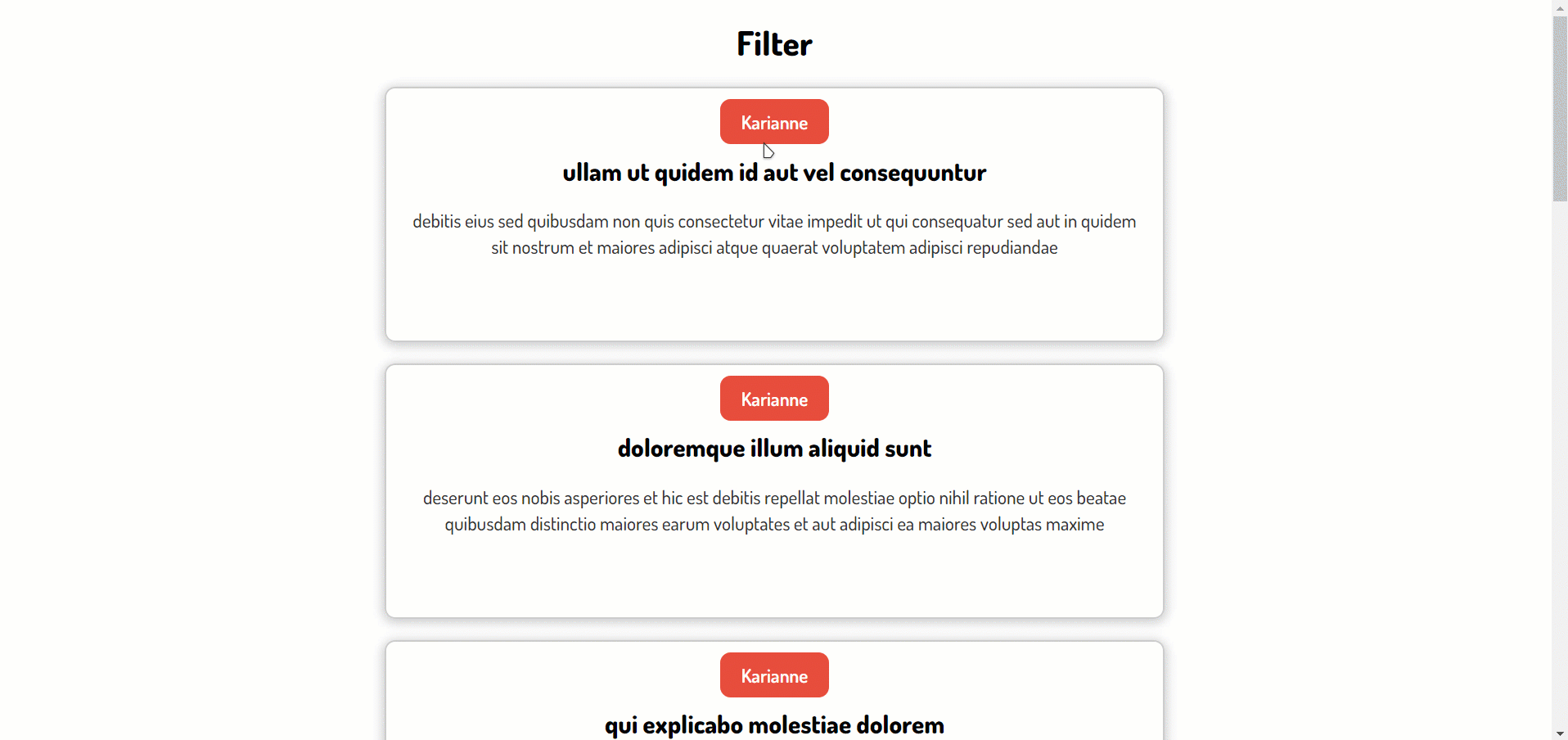
Homework
Instead of typing the username “Karianne” manually, how would it be if we created an input and created a structure that searches and filters the data and then lists it when it is typed in? Let this be your homework. ?
Browser Support
Filter method is supported by all browsers. See the table below for more detailed support information.
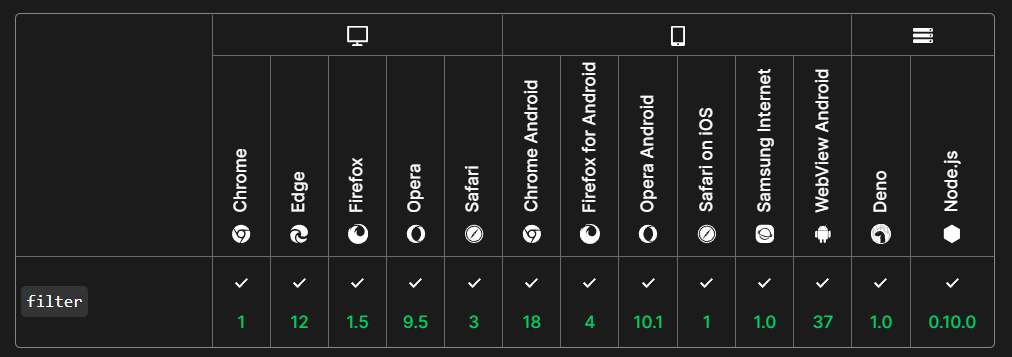
The End
Yes, we have come to the end of this blog post. In this article, we have reinforced the use of the filter method with simple examples. At the same time, we have learned how to use the filter method in daily business life with a react project example. See you in my next blog post… Take care and never forget to code. ??